In this tutorial you will learn about the C Program to Calculate Purchase Amount to be Paid after Discount and its application with practical example.
C Program to Calculate Purchase Amount to be Paid after Discount
In this tutorial, we will learn to create a C program that will Purchase Amounts to be Paid after Discount using C programming.
Prerequisites
Before starting with the tutorial we assume that you are best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
- Conditional statement in C programming.
Program to Calculate Purchase Amount to Pay after Discount
In c programming, it is possible to take numerical input from the user and Purchase Amounts to Pay after Discount with the help of a very small amount of code. The C language has many types of header libraries which has supported function in them with the help of these files the programming is easy.
Algorithm:-
With the help of this program, we can Purchase Amounts to Pay after Discount.
1 2 3 4 5 6 7 8 9 |
1. Declaring the variables for the program. 2. Taking the input amount. 3. Calculating the discount amount. 4. Calculating the gross amount. 5. Printing the gross amount of the user. |
Program:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
#include<stdio.h> int main() { //Declaring the variable float amt, discount, amtToBePaid; //Taking the input from the user printf("How much shopping amount you have done here ? "); scanf("%f", &amt); printf("\n"); //Calculating the discount if(amt<=100) printf("You have to paid: %0.2f", amt); else { if(amt>100 && amt<=200) { discount = (amt*5)/100; amtToBePaid = amt-discount; printf("After applying the discount, you have to paid: %0.2f", amtToBePaid); } else if(amt>200 && amt<=400) { discount = (amt*10)/100; amtToBePaid = amt-discount; printf("After applying the discount, you have to paid: %0.2f", amtToBePaid); } else if(amt>400 && amt<=800) { discount = (amt*20)/100; amtToBePaid = amt-discount; //Printing the discounted amount printf("After applying the discount, you have to paid: %0.2f", amtToBePaid); } else { discount = (amt*25)/100; amtToBePaid = amt-discount; //Printing the discounted amount printf("After applying the discount, you have to paid: %0.2f", amtToBePaid); } } return 0; } |
Output:-

In the above program, we have first initialized the required variable.
- amt = it will hold the float value for the basic amount.
- discount = it will hold the float value for the discount amount.
- amtToBePaid = it will hold the float value for the gross amount.
Input amount from the user.
Calculating the discount.
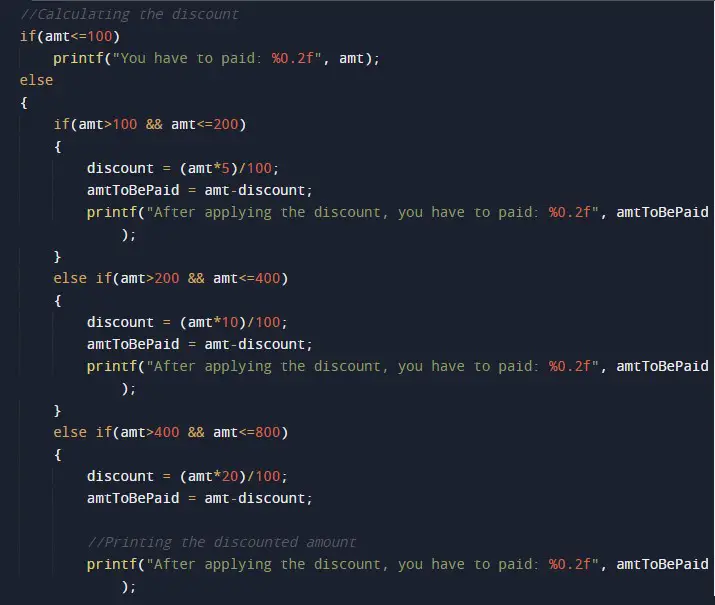
Printing amount after giving the discount.