In this tutorial you will learn about the C Program to Check Anagram or Not and its application with practical example.
C Program to Check Anagram or Not
In this tutorial, we will learn to create a C program that will Check Anagram or Not in C programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
- For loop in c programming.
- Conditional statements in C programming.
- String functions of c programming.
Program to Check Anagram or Not
As we all know the String is a collection of character data types. In strings, only one variable is declared which can store multiple values. First, we will take the input string from the user.
Then we will sort that string using the for a loop.
With the help of this program, we can Check Anagram or Not.
Algorithm:-
1 2 3 4 5 6 7 8 9 10 11 |
1. Declare the variables for the program. 2. Take the input string from the user. 3. Checking length of both the strings. 4. Sorting the string order and comparing the strings. 5. Print the output. 6. End the program. |
Program to Check Anagram or Not:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
#include <stdio.h> #include <string.h> int main (void) { // Declaring the variables. char str1[] = "recitals"; char str2[] = "articles"; char temp; int i, j; // Using string functions int n = strlen(str1); int n1 = strlen(str2); // If both strings are of different length, then they are not anagrams if( n != n1) { printf("%s and %s are not anagrams! \n", str1, str2); return 0; } // sorting both the strings first to compare − for (i = 0; i < n-1; i++) { for (j = i+1; j < n; j++) { if (str1[i] > str1[j]) { temp = str1[i]; str1[i] = str1[j]; str1[j] = temp; } if (str2[i] > str2[j]) { temp = str2[i]; str2[i] = str2[j]; str2[j] = temp; } } } // Comparing both strings character by character for(i = 0; i<n; i++) { if(str1[i] != str2[i]) { printf("Strings are not anagrams! \n", str1, str2); return 0; } } printf("Strings are anagrams! \n"); return 0; } |
Output:-
In the above program, we have first initialized the required variable.
- str1[] = it will hold the string value.
- str2[] = it will hold the string value.
- temp = it will hold the string value.
- i = it will hold the integer value.
- j = it will hold the integer value.
- n1 = it will hold the integer value.
- n2= it will hold the integer value.
Using string functions to get the length of both the strings.


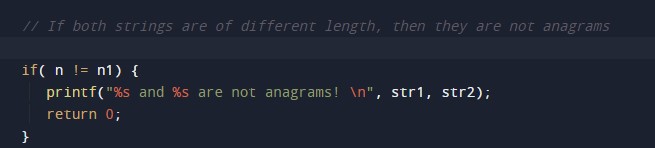
Sorting both the strings.
Comparing both the strings after Sorting.
Printing the output.