In this tutorial you will learn about the C Program to Convert Hexadecimal to Decimal and its application with practical example.
C Program to Convert Hexadecimal to Decimal
In this tutorial, we will learn to create a C program that will Convert Hexadecimal to Decimal in C programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
Program to Convert Hexadecimal to Decimal:-
As we all know the c is a very powerful language. With the help of c programming language, we can make many programs. We cal perform many input-output operations using c programming. In today’s tutorial, we take the input in hexadecimal from the user and convert it into Decimal. With the help of c programming, we can perform many conversion operations.
With the help of this program, we can Convert Hexadecimal to Decimal.
Algorithm:-
1 2 3 4 5 6 7 8 9 10 11 |
1. Declare the variables for the program. 2. Takeing the input number from from the user in hexa decimal for the program. 3. Passing that input to the string function. 4. Pass that number to a for loop for convertion. 4. Print the Result. 5. End the program. |
Program to Convert Hexadecimal to Decimal:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* C Program to Convert Hexadecimal to DECIMAL */ #include <stdio.h> #include <math.h> #include <string.h> #define ARRAY_SIZE 20 int main() { /* Declaring the variables for the program */ char hex[ARRAY_SIZE]; long long decimal = 0, base = 1; int i = 0, value, length; /* Get hexadecimal value from user */ printf("Enter hexadecimal number: "); fflush(stdin); fgets(hex,ARRAY_SIZE,stdin); length = strlen(hex); /* Converting the Hexa decimal to the decimal */ for(i = length--; i >= 0; i--) { if(hex[i] >= '0' && hex[i] <= '9') { decimal += (hex[i] - 48) * base; base *= 16; } else if(hex[i] >= 'A' && hex[i] <= 'F') { decimal += (hex[i] - 55) * base; base *= 16; } else if(hex[i] >= 'a' && hex[i] <= 'f') { decimal += (hex[i] - 87) * base; base *= 16; } } //PRINTING THE HEXADECIMAL NUMBER TO THE USER printf("\nHexadecimal number = %s", hex); //PRINTING THE DECIMAL NUMBER TO THE USER printf("Decimal number = %lld\n", decimal); return 0; } |
Output:-
In the above program, we have first initialized the required variable.

- hex[] = it will hold the Hexa value for the program.
- i = it will hold the integer value.
Taking the input.

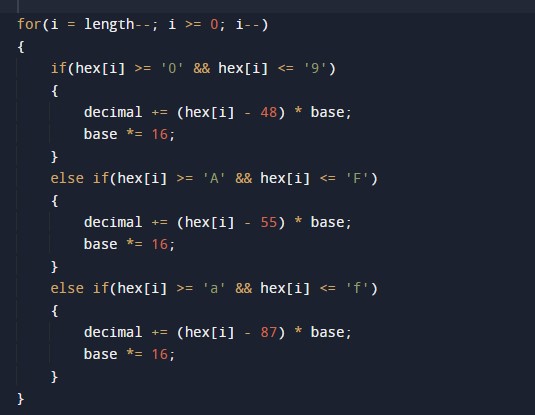