In this tutorial you will learn about the C Program to Convert Hexadecimal to Octal and its application with practical example.
C Program to Convert Hexadecimal to Octal
In this tutorial, we will learn to create a C program that will Convert Hexadecimal to Octal in C programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
Program to Convert Hexadecimal to Octal:-
As we all know the c is a very powerful language. With the help of c programming language, we can make many programs. We cal perform many input-output operations using c programming. In today’s tutorial, we take the input in hexadecimal from the user and convert it into octal. With the help of c programming, we can perform many conversion operations.
With the help of this program, we can Convert Hexadecimal to Octal.
Algorithm:-
1 2 3 4 5 6 7 8 9 |
1. Declare the variables for the program. 2. Takeing the input number from in hexa for the program. 3. Passing that input to functions. 4. Print the Result. 5. End the program. |
Program to Convert Hexadecimal to Octal:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 |
/* C Program to Convert Hexadecimal to Octal */ #include <math.h> #include <stdio.h> #include <string.h> // Function to convert HexaDecimal to Binary long long int hex_to_bin(char hex[]) { long long int bin, place; int i = 0, rem, val; bin = 0ll; place = 0ll; // Hexadecimal to binary conversion for (i = 0; hex[i] != '\0'; i++) { bin = bin * place; switch (hex[i]) { case '0': bin += 0; break; case '1': bin += 1; break; case '2': bin += 10; break; case '3': bin += 11; break; case '4': bin += 100; break; case '5': bin += 101; break; case '6': bin += 110; break; case '7': bin += 111; break; case '8': bin += 1000; break; case '9': bin += 1001; break; case 'a': case 'A': bin += 1010; break; case 'b': case 'B': bin += 1011; break; case 'c': case 'C': bin += 1100; break; case 'd': case 'D': bin += 1101; break; case 'e': case 'E': bin += 1110; break; case 'f': case 'F': bin += 1111; break; default: printf("Invalid hexadecimal input."); } place = 10000; } return bin; } // Function to convert Binary to Octal long long int bin_to_oct(long long bin) { long long int octal, place; int i = 0, rem, val; octal = 0ll; place = 0ll; place = 1; // Binary to octal conversion while (bin > 0) { rem = bin % 1000; switch (rem) { case 0: val = 0; break; case 1: val = 1; break; case 10: val = 2; break; case 11: val = 3; break; case 100: val = 4; break; case 101: val = 5; break; case 110: val = 6; break; case 111: val = 7; break; } octal = (val * place) + octal; bin /= 1000; place *= 10; } return octal; } // Function to Convert // Hexadecimal Number to Octal Number long long int hex_to_oct(char hex[]) { long long int octal, bin; // convert HexaDecimal to Binary bin = hex_to_bin(hex); // convert Binary to Octal octal = bin_to_oct(bin); return octal; } int main() { // Get the hexadecimal number char hex[20] = "1AC"; // convert hexadecimal to octal printf("Equivalent Octal Value = %lld", hex_to_oct(hex)); return 0; } |
Output:-
In the above program, we have first initialized the required variable.

- hex[20] = it will hold the Hexa value for the program.
Hexa to binary
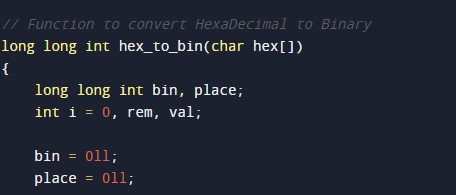
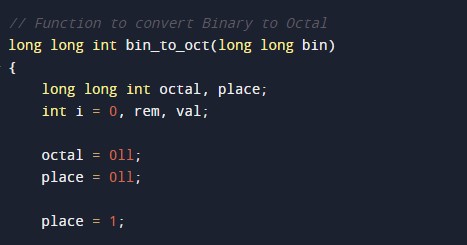
Main function.