In this tutorial you will learn about the C Program to convert Kilometer to Meter Centimeter and Millimeter and its application with practical example.
C Program to convert Kilometer to Meter Centimeter and Millimeter
In this tutorial, we will learn to create a C program that will convert the Kilometer to Meter, Centimeter, and Millimeter in C programming.
Prerequisites
Before starting with this tutorial, we assume that you are the best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C Programming.
- Arithmetic Operators in C Programming.
What are Kilometer to Meter, Centimeter and Millimeter?
The kilometers, meters, centimeters & millimeters are the metric units for the measurement of length between two points.
Description Table below.
1 2 3 4 5 |
<strong>1. 1 kilometer is equal to 1,000 Meters. 2. 1 Meter is equal to 100 Centimeters. 3. 1 Centimeters is equal to 10 Millimeters.</strong> |
Algorithm:-
1 2 3 4 5 6 7 8 9 10 11 |
1. Declaring the variable for taking the input from the user. 2. Taking the input of kilometers from the user. 3. Passing that kilometer to program code to convert. 4. Converting the units in the subunits. 5. Printing the converted values. 6. End Program. |
Program to convert the kilometers into small units.
In today’s program, we will first take the input from the user in kilometers. And then we will convert that input into meters, centimeters, and millimeters,
With the help of the below program, we can convert the kilometers to subunits.
Program Code:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
/* C Program to Convert kilometers to meters centimeters and millimeters */ #include <stdio.h> int main() { //Declaring the required variables for the program. float mm, cm, m, km; //Taking the input length from user in kilometers. printf("\n Please Enter the Length in kilometers (km) : "); scanf("%f", &km); //Converting the kilometers to meters m = km * 1000.0; //Converting the kilometers to centimeters cm = km * 100000.0; //Converting the kilometers to millimeters mm = km * 1000000.0; //printing the converted output in meters printf("\n %.2f kilometers = %.2f meters", km, m); //printing the converted output in centimeters printf("\n %.2f kilometers = %.2f centimeters", km, cm); //printing the converted output in millimeters printf("\n %.2f kilometers = %.2f millimeters", km, mm); return 0; } |
Output:-
In the above program, we have first initialized the required variable.

- km = it will store the input kilometers value.
Taking the input from the user in kilometers.

Converting into smaller values.
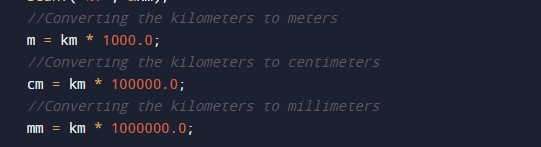
Printing the output of the program.