In this tutorial you will learn about the C Program to Multiply Two Matrices and its application with practical example.
C Program to Print Multiply Two Matrices
In this tutorial, we will learn to create a C program that will Print Multiply Two Matrices C programming.
Prerequisites
Before starting with the tutorial we assume that you are best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
- For loop in C programming.
Program to Print Multiply Two Matrices
In c programming, it is possible to take a numerical input for the size of the matrix and the elements of the matrix from the user and Print Multiply Two Matrices with the help of a very small amount of code. The C language has many types of header libraries which has supported function in them with the help of these files the programming is easy.
Algorithm:-
With the help of this program, we can Print Multiply Two Matrices.
1 2 3 4 5 6 7 8 9 10 11 |
1. Declaring the variables for the program. 2. Taking the input size of matrix. 3. Taking the input elements of matrix. 4. Calculating the<strong> Multiply Two Matrices</strong>. 5. Printing the calculated elements. 6. End Program. |
Program:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
/* C Program to Multiply Two Matrices */ #include<stdio.h> #include<stdlib.h> int main() { //declaring the variable for the program int a[10][10],b[10][10],mul[10][10],r,c,i,j,k; //Taking the input from the user printf("enter the number of row="); scanf("%d",&r); printf("enter the number of column="); scanf("%d",&c); //taking input elements of matrices printf("enter the first matrix element=\n"); for(i=0;i<r;i++) { for(j=0;j<c;j++) { scanf("%d",&a[i][j]); } } printf("enter the second matrix element=\n"); for(i=0;i<r;i++) { for(j=0;j<c;j++) { scanf("%d",&b[i][j]); } } printf("multiply of the matrix=\n"); for(i=0;i<r;i++) { for(j=0;j<c;j++) { mul[i][j]=0; for(k=0;k<c;k++) { mul[i][j]+=a[i][k]*b[k][j]; } } } //for printing result for(i=0;i<r;i++) { for(j=0;j<c;j++) { printf("%d\t",mul[i][j]); } printf("\n"); } return 0; } |
Output:-
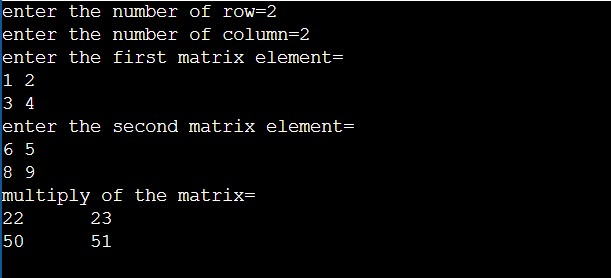
In the above program, we have first initialized the required variable.
- a[10][10]= it will hold the integer value.
- b[10][10]= it will hold the integer value.
- mul[10][10]= it will hold the integer value.
- r= it will hold the integer value.
- c= it will hold the integer value.
- k= it will hold the integer value.
- i= it will hold the integer value.
- j= it will hold the integer value.
Taking the size of the matrix from the user.
Input elements of both the matrices.
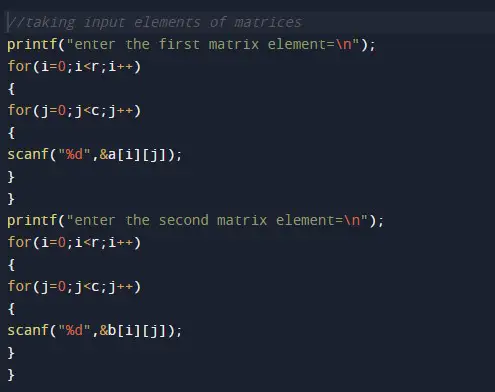
Calculating the multiply of the two matrices.
Printing the output for the program.