In this tutorial you will learn about the C Program to Print Prime Numbers and its application with practical example.
C Program to Print Prime Numbers
In this tutorial, we will learn to create a C program that will Print Prime Numbers using C programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C programming topics:
- Operators in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
- For loop in C programming.
- Conditional Statements in C programming.
- Arithmetic operations in C Programming.
Program to Print Prime Numbers
In c programming, it is possible to take numerical input from the user and check if that number is Prime Number or not with the help of a very small amount of code. The prime number means a number that is only divisible by itself. The C language has many types of header libraries that have supported functions in them with the help of these files the programming is easy.
With the help of this program, we can Print Prime Numbers.
Algorithm:-
1 2 3 4 5 6 7 8 9 |
1. Declaring the variables for the program. 2. Taking the input number from the user. 3. Calculating the number is a prime number or not. 4. Printing the result. 5. End the program. |
Program to Print Prime Numbers:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include <stdio.h> int main() { //declaring the variables for the program int no, i, flag = 0; //i for controlling the loop //no for taking the input number from the user //flag to store the temporary value for the program //taking input from the user for checking printf("Enter any positive integer: "); scanf("%d", &no); //For loop to check the number with conditions for (i = 2; i <= no / 2; ++i) { // condition for non-prime if (no % i == 0) { flag = 1; break; } } //printing the output for the given numbers. if (no == 1) { //zero is not considered as a prime number printf("1 is neither prime nor composite."); } else { if (flag == 0) //if the number is a prime number then this message is shown printf("%d is a prime number.", no); else //else not a prime number is shown printf("%d is not a prime number.", no); } return 0; } |
Output:-
In the above program, we have first initialized the required variable.
- no= it will hold the integer value of the input.
- i = it will hold the integer value.
- flag = it will hold the integer value.
Input message for the user for the integer value.
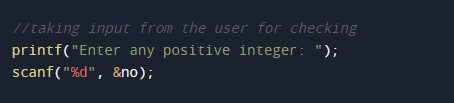
Program Logic Code.

Printing output prime number or not.