In this tutorial you will learn about the C Program to Reverse a String and its application with practical example.
C Program to Reverse a String
In this tutorial, we will learn to create a C program that will Reverse a String in C programming.
Prerequisites
Before starting with this tutorial, we assume that you are the best aware of the following C programming topics:
- Operators in C Programming.
- Usage of Conditional statements in C Programming.
- Basic Input and Output function in C Programming.
- Basic C programming.
- For loop in C Programming.
What is reversing a string?
A string is a collection of words, numbers, and symbols. The String can be any sentence of English Language. Reversing a string means writing the sentence or a string in reverse order from last to first.
Algorithm:-
1 2 3 4 5 6 7 8 9 10 11 |
1. Declaring the variables for the program. 2. Taking the input string from the user. 3. Sending that statement to the for loop for reversal. 4. Passing that reversed string to the user. 5. Printing the result string. 6. End the prorgam. |
Program Description for Reversing the String:-
In this program, We will first take the input string from the user in string format. Then we will find the length of that string using strlen(); after that reversing that string using the for-loop. Storing the reversed sentence in a variable. Printing the reversed string from the program.
With the help of the below, code we will Reverse the String.
Program Code:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
/* C Program to Reverse a String */ #include <stdio.h> #include <string.h> // function definition of the revstr() void revstr(char *str1) { //declaring the required variables for the program int i, len, flag; //str = it will hold the input string. //i = it will hold the integer value for the loop. //flag = it will hold the temporary value. //len = it will hold the integer value for the length of string len = strlen(str1); // use strlen() to get the length of str string // use for loop to iterate the string for (i = 0; i < len/2; i++) { // flag variable use to temporary hold the string flag = str1[i]; str1[i] = str1[len - i - 1]; str1[len - i - 1] = flag; } } int main() { char str[50]; // size of char string //Taking the input string from the user. printf (" Enter the string: "); gets(str); // use gets() function to take string printf (" \n Before reversing the string: %s \n", str); // call revstr() function revstr(str); printf (" After reversing the string: %s", str); } |
Output:-
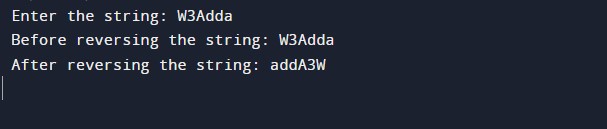
In the above program, we have first initialized the required variable.

- str = string type variable that will hold the value of the input sentence.
- i = it will hold the integer value.
- len = it will hold the integer value.
- flag = it will hold the temporary integer value.
Input string from the user for the program.
Reverse The string.
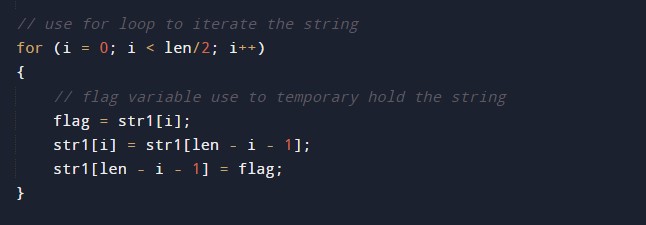
Printing the reversed string.