In this tutorial you will learn about the C++ Program to Demonstrate Operator Overloading and its application with practical example.
C++ Program to Demonstrate Operator Overloading
In this tutorial, we will learn to create a C++ program that will Demonstrate Operator Overloading in C++ programming.
Prerequisites.
Before starting with this tutorial we assume that you are best aware of the following C++ programming topics:
- Operators in C++ Programming.
- Basic Input and Output function in C++ Programming.
- Basic C++ programming.
- Classes and Objects in C++ programming.
What are the operators?
Operators are symbols with special meanings in a programming language for datatypes. The operators are used between one or more operands to perform the operations.
There are three types of operators
1 2 3 4 5 6 7 8 |
Operators in C++ can be categorized into 6 types: 1. Arithmetic Operators 2. Assignment Operators 3. Relational Operators 4. Logical Operators 5. Bitwise Operators 6. Other Operators |
In this program, we will overload the Arithmetic operator i.e. “++”.
Algorithm:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
1. Starting the program. 2. Declaring the class that will overload the operator. 3. Declaring the variable required for the program. 4. Creating a Constructor to initialize count to 8. 5. Overloading the ++ when used as prefix. 6. Call the "void operator ++ ()" function. 7. Printing the data afteroverloading the ++ operator. 7. End program. |
Program for Operator overloading:-
In this program, we will Overload the increment operator. We will use the operators to work for user-defined classes. It means we will use the operators with special meaning in the program for a data type.
Program to overload increment operator:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// Overload ++ when used as prefix #include <iostream> using namespace std; //declaring the class that will overload the operator class Count { //declaring the variable required for the program private: int i; public: // Creating a Constructor to initialize count to 8 Count() : i(8) {} // Overloading the ++ when used as prefix void operator ++ () { ++i; } //printing the data afteroverloading the ++ operator void display() { cout << "Count: " << i << endl; } }; int main() { Count count1; // Call the "void operator ++ ()" function ++count1; count1.display(); return 0; } |
Output for increment:-
Declaring the required variables for the program.



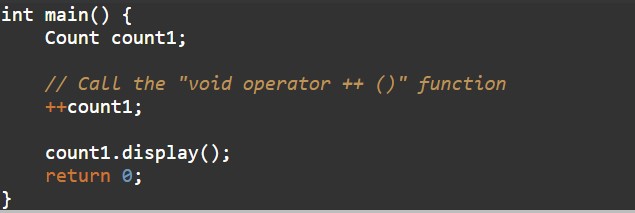
