In this tutorial you will learn about the c++ program to merge two arrays and its application with practical example.
C++ Program to Merge Two Arrays
In this tutorial, we will learn to create a C++ program that will merge two arrays using C++ programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C++ programming topics:
- Operators in C++ Programming.
- Basic Input and Output function in C++ Programming.
- Basic C++ programming.
- For loop in C++ Programming.
Algorithm:-
1 2 3 4 5 6 7 8 9 |
1. Declaring the variables and arrays. 2. Taking input in Array. 3. Merging the arrays into a single array. 4. Printing the merged array. 5. End Program. |
Merging of two arrays:-
In Today’s we will merge two different arrays into a single array with a very simple and shortcode. First, we will take two arrays in input and then we will merge them into one single array using short C++ programming.
Program:-
To find Merge the two array elements into a single array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
// C++ program to merge two sorted arrays/ #include<iostream> using namespace std; void mergeArrays(int arr1[], int arr2[], int n1, int n2, int arr3[]) { int i = 0, j = 0, k = 0; // Traverse both array while (i<n1 && j <n2) { // Check if current element of first // array is smaller than current element // of second array. If yes, store first // array element and increment first array // index. Otherwise do same with second array if (arr1[i] < arr2[j]) arr3[k++] = arr1[i++]; else arr3[k++] = arr2[j++]; } // Store remaining elements of first array while (i < n1) arr3[k++] = arr1[i++]; // Store remaining elements of second array while (j < n2) arr3[k++] = arr2[j++]; } //main function code int main() { //declaring the variables for the program int arr1[] = {1, 3, 5, 7}; int n1 = sizeof(arr1) / sizeof(arr1[0]); int arr2[] = {2, 4, 6, 8}; int n2 = sizeof(arr2) / sizeof(arr2[0]); int arr3[n1+n2]; mergeArrays(arr1, arr2, n1, n2, arr3); cout << "Array after merging" <<endl; for (int i=0; i < n1+n2; i++) cout << arr3[i] << " "; return 0; } |
Output:-
In the above program, we have first initialized the required variable
- arr1[] = it will hold the elements in an array1.
- arr2[] = it will hold the elements in an array2.
- arr3[] = it will hold the elements in an array3.
- mergeArrays[] = it will hold the elements in an array.
- i = it will hold the integer value to control array using for loop.
- j = it will hold the integer value to control array using for loop.
- k = it will hold the integer value to control array using for loop.
Taking input for array1 as well as array2.
Merging the two arrays.
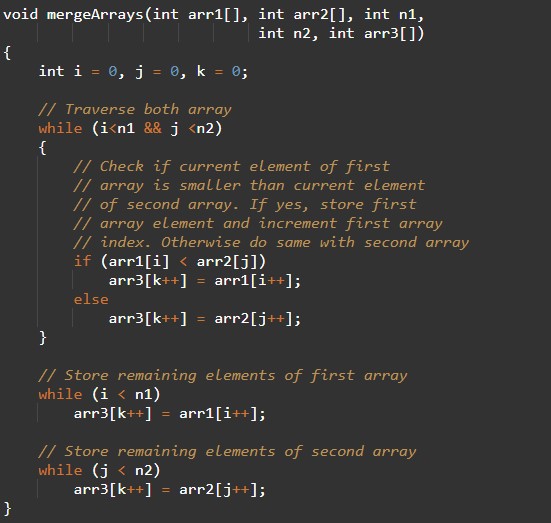