In this tutorial you will learn about the C++ Program to Remove all Characters in a String Except Alphabets and its application with practical example.
C++ Program to Remove all Characters in a String Except Alphabets
In this tutorial, we will learn to create a C++ program that will Remove all Characters in a String Except Alphabets in C++ programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C++ programming topics:
- Operators in C++ Programming.
- Basic Input and Output function in C++ Programming.
- Basic C++ programming.
- For loop in C++ programming.
- Conditional statements in C++ programming.
- String functions of C++ programming.
What are Characters in a String?
The characters can be anything typed on the screen i.e. letter or number in a string. The string is a collection of characters. In C++ we can take input string from the user and store it in a variable.
Algorithm:-
1 2 3 4 5 6 7 8 9 10 11 |
1. Start Program. 2. Declaring the required variables for the program. 3. Taking input string from the user for the program. 4. Passing that string to for loop for removing the data. 5. Printing the output string to user. 6. End Program. |
Program to Remove all Characters in a String Except Alphabets:-
In today’s program, we will take the input string from the user and store it in a variable then we will remove all Characters in a String Except Alphabets. After removing we will print that string on the output screen.
The below will be an example program to remove the characters from the string.
Program:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
/* C++ Program to Remove all Characters in a String Except Alphabets */ #include <iostream> using namespace std; int main() { //Declaring the required variables for the program. string str; string flag = ""; //Taking the input string from the user for program cout << "Enter the string value: "; getline(cin, str); //Passing that string to for loop for removing the data for (int i = 0; i < str.size(); ++i) { if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z')) { flag = flag + str[i]; } } str = flag; //Printing the output string to user. cout << "Output String: " << str; return 0; } |
Output:-
In the above program, we have first initialized the required variable.
- str = it will hold the string value.
- flag = it will hold the string value.
Taking the input string from the user.

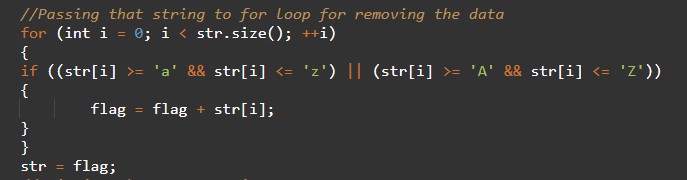