In this tutorial you will learn about the C++ Programs to Check Given Number is Palindrome or not and its application with practical example.
C++ Programs to Check Given Number is Palindrome or not
In this tutorial, we will learn to create a C++ program that will Check Given Number is Palindrome or not using C++ programming.
Prerequisites
Before starting with this tutorial we assume that you are best aware of the following C++ programming topics:
- Operators in C++ Programming.
- Basic Input and Output function in C++ Programming.
- Basic C++ programming.
- For loop in C++ programming.
- Conditional Statements in C++ programming.
- Arithmetic operations in C++ Programming.
What is a palindrome number?
The Palindrome number means a number that is the same when we read it from forward and reverse.
For example:-
- 1221 this is a palindrome number is the same when we write it in reverse order 1221.
- 123 this is not a palindrome number it is not the same when we write it in reverse order 321.
Algorithm:-
1 2 3 4 5 6 7 8 9 |
1. Declaring the variables for the program. 2. Taking the input number from the user. 3. Calculating the number is a Palindrome number or not. 4. Printing the result. 5. End the program. |
Program to Check Whether a Number is Palindrome or Not:-
To check whether a number is a Palindrome Number or not. In today’s C++ program we will write code for checking. First, we will take a number in input and then pass it into the program to Check Whether a Number is Palindrome or Not.
With the help of the below program, we can Check Palindrome or Not.
Program Code:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include <iostream> using namespace std; int main() { //Declaring the variables for the program. int n, num, digit, rev = 0; //Taking the input from user positive integer value cout << "Enter a positive number: "; cin >> num; n = num; //Checking the palindrome numbers do { digit = num % 10; rev = (rev * 10) + digit; num = num / 10; } while (num != 0); //Printing the output checked number is palindrome or not. cout << " The reverse of the number is: " << rev << endl; if (n == rev) cout << " The number is a palindrome."; else cout << " The number is not a palindrome."; return 0; } |
Output:-
In the above program, we have first initialized the required variable.
- num= it will hold the integer value of the input.
- n = it will hold the integer value.
- digit = it will hold the integer value.
- rev = it will hold the integer value.
Input message for the user for the integer value.


Program Logic Code.
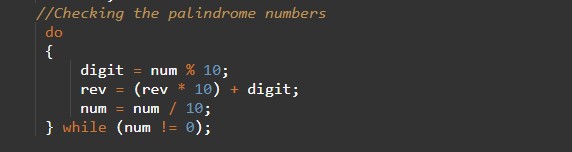
Printing output Palindrome number or not.