In this tutorial you will learn about the Java Program to Multiply two Numbers and its application with practical example.
Java Program to Multiply Two Numbers
In this tutorial, we will learn to create a Java program that will Multiply Two Numbers in Java programming.
Prerequisites
Before starting with this tutorial, we assume that you are the best aware of the following Java programming topics:
- Operators in Java Programming.
- Basic Input and Output function in Java Programming.
- Basic Java Programming.
- Arithmetic operations in java programming.
Program to Multiply Two Numbers:-
In today’s tutorial, we will create a program that will multiply the two numbers using the concepts of java programming. First, we will take the two numbers in input from the user. Then we will multiply the two numbers from the code. At last, we will print the output of the program.
With the help of this program, we can take input and Multiply Two Numbers.
Algorithm to multiply the numbers:-
1 2 3 4 5 6 7 8 9 10 11 |
1. First start the main function body. 2. Declaring the variables for the program. 3. Taking the input numbers from the user. 4. Converting that number to an integer. 5. Multiply the numbers and storing it in a variable. 6. End the program. |
Program to Multiply Two Numbers:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
// Java Program to Multiply two Numbers import java.util.*; public class Multiply { public static void main(String args[]) { //Declaring the required variables for the program int no1, no2, multiply; //Taking the input numbers from the user. Scanner buf = new Scanner(System.in); //Taking the first number System.out.print("Enter first number : "); no1 = buf.nextInt(); //Taking the second number System.out.print("Enter second number : "); no2 = buf.nextInt(); /*Calculating the multiplication of two numbers.*/ multiply = no1 * no2; //Printing the multiplication of two numbers.. System.out.print("Multiply of two numbers is : " + multiply); } } |
Output:-
In the above program, we have first initialized the required variable.

- no1 = it will hold the input number value from the user.
- no2 = it will hold the input number value from the user.
- Multiply = it will hold the sum value of the numbers.
Taking input numbers from the user.
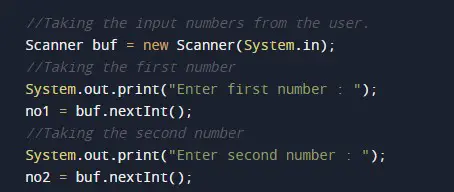
Calculating the multiply of two numbers.
Printing the output.
