In this tutorial you will learn about the Java Program to Print 1 to 100 Without Loop and its application with practical example.
Java Program to Print 1 to 100 without using Loop
In this tutorial, we will learn to create a Java program that will Print 1 to 100 without using Loop in Java programming.
Prerequisites
Before starting with this tutorial, we assume that you are the best aware of the following Java programming topics:
- Operators in Java Programming.
- Basic Input and Output function in Java Programming.
- Basic Java programming.
- Conditional Statements in Java programming.
Algorithm:-
1 2 3 4 5 6 7 8 9 |
1. Declaring the main function. 2. Defining the initial value. 3. Calling the user defined function and passing the value. 4. Printing the series from the function. 5. End the program. |
Print 1 to 100 without using Loop:-
As we all know, the series printing works are done using the loops, but today we will print a series from 1 to 100 without using Loop. In this program, we will first define the variables for the program. Then we will pass that variable to a user-defined function to print the series. The function first prints a number and increases the value by 1.
With Java programming, we can make many arithmetic series with just a little code.
With the help of this program, we can Print 1 to 100 without using Loop.
Program:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Sample Java Program to Print 1 to 100 without Loop import java.util.*;//Importing the packages of java public class Print1to100 { //Body of the main function public static void main(String[] args) { int number = 1; printNumbers(number); } //Body of the user defined function public static void printNumbers(int num) { //Checkinf the condition for the funtion processing if(num <= 100) { //Printing the output number to the user by the program. System.out.print(num +" "); //Increasing the value of number by 1 printNumbers(num + 1); } } } |
Output:-
In the above program, we have first initialized the required variable.

- number = it will hold the integer value for the program.
Sending the data to the user-defined function for the generation of series.

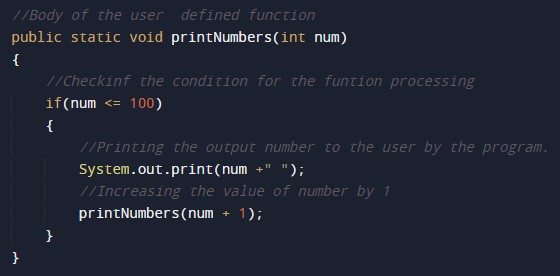
Program code to print the list up to the given number 100.